I wanted to learn reactjs so I went to the ReactJs website, did the tutorial and was ready to go. After hours of research it became quite apparent that there weren't many blogs detailing the steps for introducing reactjs into an existing asp.net core website.
So here's my attempt to help. (I'm also using Razor Pages in my application) I'm running the following application versions:
- ASP.NET Core 3
- React JS 16.13.1
- Visual Studio Code 1.44.0
- Typescript 3.8.3
On the page you want to introduce reactjs add a div with an id for reactjs to hook onto, e.g.
<div id="content"/>
You'll also need to add some script tags on that same page which reference the react libraries & the script file that will contain all of the custom components created for the application.
<script crossorigin src="https://unpkg.com/react/umd/react.development.js"></script>
<script crossorigin src="https://unpkg.com/react-dom/umd/react-dom.development.js"></script>
<script src="~/dist/main.js"></script>
Create the following folder and file structure, reactjs.cshtml is the razor file which will introduce the reactjs code.
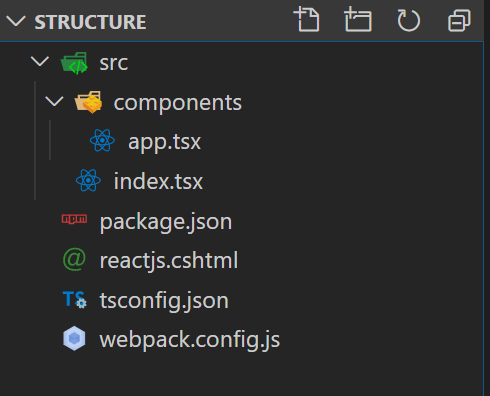
Open a command line tool at the location of the razor file where you want to introduce reactjs and run the following commands:
npm init -y
npm install --save-dev webpack webpack-cli
npm install --save react react-dom
npm install --save-dev @types/react @types/react-dom
npm install --save-dev typescript ts-loader source-map-loader
These commands do the following:
- Initialise an empty nodejs package
- Install webpack package - a tool used for bundling and minification of javascript (et al.)
- Install react packages
- Install react typescript types - this allows working with Typescript and reactjs
- Install typescript loaders - this allows webpack to transpile typescript files and convert them into javascript
Add the following to the tsconfig.json: (make sure you update [PATH-TO-YOUR-wwwroot-FOLDER])
{
"compilerOptions": {
"outDir": "[PATH-TO-YOUR-wwwroot-FOLDER]/dist",
"sourceMap": true,
"noImplicitAny": true,
"module": "commonjs",
"target": "es6",
"jsx": "react"
}
}
Add the following to the webpack.config.js: (make sure you update [PATH-TO-YOUR-wwwroot-FOLDER])
const path = require("path");
module.exports = {
mode: "production",
devtool: "source-map",
resolve: {
extensions: [".ts", ".tsx"]
},
output: {
path: path.resolve(__dirname, "[PATH-TO-YOUR-wwwroot-FOLDER]/dist"),
filename: "[name].js"
},
module: {
rules: [
{
test: /\.ts(x?)$/,
exclude: /node_modules/,
use: [
{
loader: "ts-loader"
}
]
},
{
enforce: "pre",
test: /\.js$/,
loader: "source-map-loader"
}
]
},
externals: {
"react": "React",
"react-dom": "ReactDOM",
"react-router-dom": "ReactRouterDOM"
}
};
Add the following to the index.tsx:
import * as React from "react";
import * as ReactDOM from "react-dom";
import { App } from "./components/App";
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById("content")
);
Add the following to the app.tsx:
import * as React from "react";
export class App extends React.Component<{}, {}> {
render() {
return (
<div>Hola</div>
);
}
}
The project is now setup ready for reactjs run the following command:
npm webpack
This will bundle the react scripts and output them in the wwwroot folder. You can now run your application as usual and navigate to that page.