Below shows a code example of how to covert a certificate to a Base64 string in order to create a point to site VPN for use with Azure.
1. Create a certificate
The makecert command is only available in the Visual Studio command prompt. This location varies depending on the version of Visual Studio, for VS 2017 community the location is here 'C:\Program Files (x86)\Microsoft Visual Studio\2017\Community\Common7\Tools\VsDevCmd.bat'
cd D:\test
makecert -n "CN=RootCertificate" -r -a sha256 -sv RootCertificate.pvk "RootCertificate.cer"
The private key RootCertificate.pvk can be removed from the above, however it is useful when creating client certificates from this root.
If you have left the private key in you should see the following:
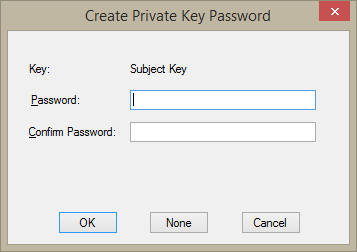
Click none for the basis of this test
2. Convert the certificate to a Base64 string
The rest of these steps require powershell
function Create-Base64Certificate {
$CertificatePath = "D:\test\RootCertificate.cer"
$certificate = new-object System.Security.Cryptography.X509Certificates.X509Certificate2($CertificatePath)
$certificateBase64 = [system.convert]::ToBase64String($certificate.RawData)
$certificateBase64 >> out.txt
}
Create-Base64Certificate
3. Review contents of out.txt
You should see something similar
MIIC7DCCAdSgAwIBAgIQc2lqZUFPUphLzpzl91Eh9jANBgkqhkiG9w0BAQsFADAPMQ0wCwYDVQQDEwRSb290MB4XDTE3MDMxNzExMzEzNloXDTM5MTIzMTIzNTk1OVowDzENMAsGA1UEAxMEUm9vdDCCASIwDQYJKoZIhvcNAQEBBQADggEPADCCAQoCggEBAJ7PDJ00JeGv9FTVqdFXt25W+zofrDg/jEWHMhmeEUZY4GS/zXHCEqZqv7hPRrgfJTiv9TeRe0Vn2tAAv1Fa207cENS4qzNAlE9zY8+VvXbuxxo5SZMiIXawGlDAX6Q3zvu0FoReczeHh6ydJcAeZRfbPt5SwiywzTtGqg/UV6RF9d416FKbqOQxX7jE1nQM2I+F8Rh3+tWPo2BJCDDCYMSKylgAmmCceBzfMWsloNPTfyJEU8AHTGRobxGeWd6yeqfX3F8z86RPlRHarY/ZOqDje+jB8J2U3QvsHgNr719TCTkHCP5lO01RiDZFIaQYdhcw/L9Or8K3ElirKyTP1yECAwEAAaNEMEIwQAYDVR0BBDkwN4AQh2KHxFYVw2aPdpwrfB06zaERMA8xDTALBgNVBAMTBFJvb3SCEHNpamVBT1KYS86c5fdRIfYwDQYJKoZIhvcNAQELBQADggEBAEmCPKG0yz5ONR3zfNXNmaxbcI6m3CEyv9mSHW1s32iRihyx2o6lkaknz8OnWsqiAVIh2V5O0A9CHESXweHx2TOeiQTLY6ViV1s73zEDJpYC1F8sulDwGRhqGa51zBEYzW4p+6ImTH4sXLdAvc/Fbj25Qy2jRdz2mgEJxlui5WVeC9PIIxdpbybfqApTNewa9rblhr5Xe/n/Kn3ETiGHmRxaPHU5jg7wsAPSI4C8mtqGGyoSf8ucglFb3WEny0vfmijVfiFL+FipfIDAFsuFOBYh4XyxvMoZZEKIHkj9bezt4u/pqhDb5Abszu8NVhClLu090zo3CifePD7/cCqlfNE=
This Base64 string can be used when creating and uploading a root certificate to an Azure VPN.
4. Try it in an Azure Template
{
"apiVersion": "2015-06-15",
"type": "Microsoft.Network/virtualNetworkGateways",
"name": "api-gateway-test",
"location": "[resourceGroup().location]",
"dependsOn": [
"[concat("Microsoft.Network/publicIPAddresses/", parameters("gatewayPublicIPName"))]",
"[concat("Microsoft.Network/virtualNetworks/", variables("virtualNetworkName"))]"
],
"properties": {
"ipConfigurations": [
{
"properties": {
"privateIPAllocationMethod": "Dynamic",
"subnet": {
"id": "[variables("gatewaySubnetRef")]"
},
"publicIPAddress": {
"id": "[resourceId("Microsoft.Network/publicIPAddresses",parameters("gatewayPublicIPName"))]"
}
},
"name": "vnetGatewayConfig"
}
],
"sku": {
"name": "Basic",
"tier": "Basic"
},
"gatewayType": "Vpn",
"vpnType": "RouteBased",
"enableBgp": "false",
"vpnClientConfiguration": {
"vpnClientAddressPool": {
"addressPrefixes": [
"192.168.1.0/26"
]
},
"vpnClientRootCertificates": [
{
"name": "TestRootCert",
"properties": {
"PublicCertData": "[parameters("certificateBase64String")]"
}
}
]
}
}
}